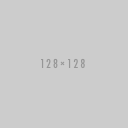
John Smith @johnsmith 31m
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Aenean efficitur sit amet massa fringilla
egestas. Nullam condimentum luctus turpis.
Modal
A classic modal overlay, in which you can include any content you want
The modal structure is very simple:
modal
: the main container
modal-background
: a transparent overlay that can act as a click target to close the modalmodal-content
: a horizontally and vertically centered container, with a maximum width of 640px,
in which you can include any content
modal-close
: a simple cross located in the top right corner
<div class="modal">
<div class="modal-background"></div>
<div class="modal-content">
<!-- Any other Bulma elements you want -->
</div>
<button class="modal-close is-large" aria-label="close"></button>
</div>
To activate the modal, just add the is-active
modifier on the
.modal
container. You may also want to add is-clipped
modifier to a containing element
(usually html
) to stop scroll overflow.
Because a modal can contain anything you want, you can very simply use it to build an image gallery for example:
<div class="modal">
<div class="modal-background"></div>
<div class="modal-content">
<p class="image is-4by3">
<img src="https://bulma.io/assets/images/placeholders/1280x960.png" alt="">
</p>
</div>
<button class="modal-close is-large" aria-label="close"></button>
</div>
If you want a more classic modal, with a head, a body and a foot,
use the modal-card
.
<div class="modal">
<div class="modal-background"></div>
<div class="modal-card">
<header class="modal-card-head">
<p class="modal-card-title">Modal title</p>
<button class="delete" aria-label="close"></button>
</header>
<section class="modal-card-body">
<!-- Content ... -->
</section>
<footer class="modal-card-foot">
<div class="buttons">
<button class="button is-success">Save changes</button>
<button class="button">Cancel</button>
</div>
</footer>
</div>
</div>
The Bulma package does not come with any JavaScript. Here is however an implementation example,
which sets the click
handlers for custom elements, in vanilla JavaScript.
There are 3 parts to this implementation:
click
event on the trigger to open the
modal
At the end of your page, before the closing </body>
tag, at the following HTML snippet:
<div id="modal-js-example" class="modal">
<div class="modal-background"></div>
<div class="modal-content">
<div class="box">
<p>Modal JS example</p>
<!-- Your content -->
</div>
</div>
<button class="modal-close is-large" aria-label="close"></button>
</div>
The id
attribute's value must be unique.
Somewhere on your page, add the following HTML button:
<button class="js-modal-trigger" data-target="modal-js-example">
Open JS example modal
</button>
You can style it however you want, as long as it has the js-modal-trigger
CSS class and the appropriate
data-target
value. For example, you can add the button is-primary
Bulma classes:
In a script
element (or in a seperate .js
file), add the following JS code:
document.addEventListener('DOMContentLoaded', () => {
// Functions to open and close a modal
function openModal($el) {
$el.classList.add('is-active');
}
function closeModal($el) {
$el.classList.remove('is-active');
}
function closeAllModals() {
(document.querySelectorAll('.modal') || []).forEach(($modal) => {
closeModal($modal);
});
}
// Add a click event on buttons to open a specific modal
(document.querySelectorAll('.js-modal-trigger') || []).forEach(($trigger) => {
const modal = $trigger.dataset.target;
const $target = document.getElementById(modal);
$trigger.addEventListener('click', () => {
openModal($target);
});
});
// Add a click event on various child elements to close the parent modal
(document.querySelectorAll('.modal-background, .modal-close, .modal-card-head .delete, .modal-card-foot .button') || []).forEach(($close) => {
const $target = $close.closest('.modal');
$close.addEventListener('click', () => {
closeModal($target);
});
});
// Add a keyboard event to close all modals
document.addEventListener('keydown', (event) => {
if(event.key === "Escape") {
closeAllModals();
}
});
});
As long as you can toggle the is-active
modifier class on the modal
element, you can
choose how you want to implement it.
Sass Variable
|
CSS Variable
|
Value
|
---|---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Modal title
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nulla accumsan, metus ultrices eleifend gravida, nulla nunc varius lectus, nec rutrum justo nibh eu lectus. Ut vulputate semper dui. Fusce erat odio, sollicitudin vel erat vel, interdum mattis neque.
Curabitur accumsan turpis pharetra augue tincidunt blandit. Quisque condimentum maximus mi, sit amet commodo arcu rutrum id. Proin pretium urna vel cursus venenatis. Suspendisse potenti. Etiam mattis sem rhoncus lacus dapibus facilisis. Donec at dignissim dui. Ut et neque nisl.
Quisque ante lacus, malesuada ac auctor vitae, congue non ante. Phasellus lacus ex, semper ac tortor nec, fringilla condimentum orci. Fusce eu rutrum tellus.
Ut venenatis, nisl scelerisque sollicitudin fermentum, quam libero hendrerit ipsum, ut blandit est tellus sit amet turpis.
Quisque at semper enim, eu hendrerit odio. Etiam auctor nisl et justo sodales elementum. Maecenas ultrices lacus quis neque consectetur, et lobortis nisi molestie.
Sed sagittis enim ac tortor maximus rutrum. Nulla facilisi. Donec mattis vulputate risus in luctus. Maecenas vestibulum interdum commodo.
Suspendisse egestas sapien non felis placerat elementum. Morbi tortor nisl, suscipit sed mi sit amet, mollis malesuada nulla. Nulla facilisi. Nullam ac erat ante.
Nulla efficitur eleifend nisi, sit amet bibendum sapien fringilla ac. Mauris euismod metus a tellus laoreet, at elementum ex efficitur.
Maecenas eleifend sollicitudin dui, faucibus sollicitudin augue cursus non. Ut finibus eleifend arcu ut vehicula. Mauris eu est maximus est porta condimentum in eu justo. Nulla id iaculis sapien.
Phasellus porttitor enim id metus volutpat ultricies. Ut nisi nunc, blandit sed dapibus at, vestibulum in felis. Etiam iaculis lorem ac nibh bibendum rhoncus. Nam interdum efficitur ligula sit amet ullamcorper. Etiam tristique, leo vitae porta faucibus, mi lacus laoreet metus, at cursus leo est vel tellus. Sed ac posuere est. Nunc ultricies nunc neque, vitae ultricies ex sodales quis. Aliquam eu nibh in libero accumsan pulvinar. Nullam nec nisl placerat, pretium metus vel, euismod ipsum. Proin tempor cursus nisl vel condimentum. Nam pharetra varius metus non pellentesque.
Aliquam sagittis rhoncus vulputate. Cras non luctus sem, sed tincidunt ligula. Vestibulum at nunc elit. Praesent aliquet ligula mi, in luctus elit volutpat porta. Phasellus molestie diam vel nisi sodales, a eleifend augue laoreet. Sed nec eleifend justo. Nam et sollicitudin odio.
Cras in nibh lacinia, venenatis nisi et, auctor urna. Donec pulvinar lacus sed diam dignissim, ut eleifend eros accumsan. Phasellus non tortor eros. Ut sed rutrum lacus. Etiam purus nunc, scelerisque quis enim vitae, malesuada ultrices turpis. Nunc vitae maximus purus, nec consectetur dui. Suspendisse euismod, elit vel rutrum commodo, ipsum tortor maximus dui, sed varius sapien odio vitae est. Etiam at cursus metus.